Goal of this exercise is to show how easy it is to deploy our python code to AWS.
Tools/prerequisites we are going to use: Visual Studio Code, NPM, python 3, AWS account, AWS credentials configured locally.
GitRepo is available here.
Serverless is a great tool to have the production code and required cloud computing configuration next to each other. To install serverless, use the following command in your favorite shell:
Note: you will need “npm” installed
npm install serverless -g
Create your folder structure for the project: we are going to need one python file, which will be the host of called function and add a file called serverless.yml, for configuration.
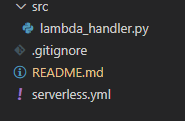
Let’s start with the python file:
import datetime
def handler(event, context) -> str:
dt = datetime.datetime.now(datetime.timezone.utc)
utc_time = dt.replace(tzinfo=datetime.timezone.utc)
return str(utc_time)
It is a pretty simple code, which will return the UTC time formatted to string. Which we need to note is the two parameters of our function: event and context, both of them will be added by AWS. As in this exercise we don’t care about input parameters, just add them to our function.
Go to the serverless.yml and add these lines:
service: BlogDavidPythonLambdaDeploy
provider:
name: aws
region: eu-west-1
functions:
utc-time-teller:
name: utc-time-teller
handler: src/lambda_handler.handler
memorySize: 128
timeout: 30
runtime: python3.8
service: is the name of the group.
provider: configuration of cloud computing platform.
name: which cloud computing platform we are using.
region: AWS’ regions are available here.
functions: in the use case of AWS, functions are the Lambdas.
“utc-time-teller”: the identifier of our Lambda.
name: how the function will be named on AWS.
handler: path to our function in the form: path/file.functionName
memorySize: how large lambda we are planning to use. Measured in MB-s. Further details and prices can be found here.
timeout: maximum processing time, measured in seconds.
runtime: container type definition with the needed prerequisites like installed python3.8. Further AWS runtimes can be found here.
If we are done with our files, open up a command line tool and call this command:
serverless deploy
Serverless will pack our code and create the stack on AWS. After it finishes, go to AWS Console and in the search bar look for “lambda”:
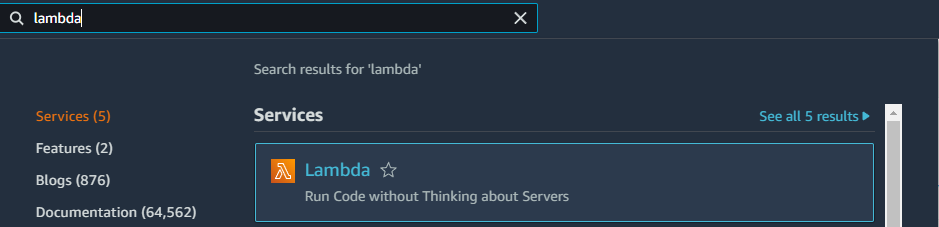
Search for the name of our lambda “utc-time-teller”:
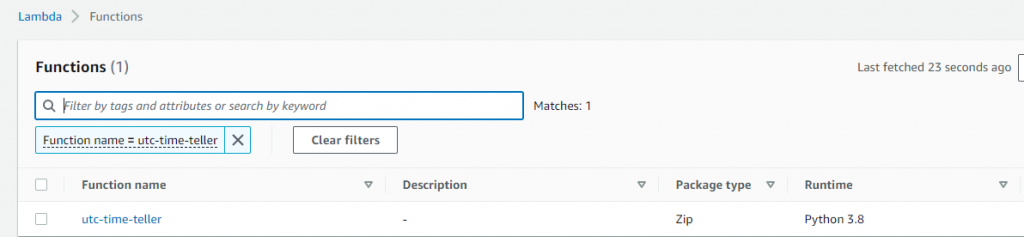
Click on it and navigate to the “Test” tab, then hit the “Test” button:

If everything went alright, we can see the result of our Lambda:

Pingback: Deploying python function as Lambda with API Gateway - Dávid's Dev Blog